String:
Examples of string operations in Python:
String Creation:
Strings can be created using single quotes ( ‘ ), double quotes ( “ ), or triple quotes ( ”’ or “””)
String Concatenation:

String Repetition:

Accessing/indexing characters :
You can access individual characters in a string by using indexing. In Python, indexing starts from 0. For example:
String Slicing:
You can extract a substring from a string by using slicing. Slicing allows you to specify a range of indices to extract. For example:
Determination of Length:
You can determine the length of a string using the len() function. For example:
Update and Delete String:
Strings are immutable which means elements of a string cannot be changed once the they are assigned with any values. For example:
- If we want to change elements of my_str1[-4:] from “Rana” to “Masud” , it will show error.
- If we want to delete elements of my_str1[0:2] , it will show error.
- If we want to delete complete string by del, it will delete entire variable
String Membership:
String membership refers to checking whether a substring or a specific character is present in a given string.
You can use the in keyword to check if a substring or character is present in a string. Output will be True if substring or character is present and output will be False in case of absence
You can also use the not in keyword to check if a substring or character is not present in a string. Output will be True if substring or character is not present and output will be False in case of presence.
Here are some examples:
String Partitioning:
String partitioning refers to splitting a string into three parts based on a specified separator. The partition() method is used for this purpose. It returns a tuple containing three elements: the part before the separator, the separator itself, and the part after the separator.
Basic syntax: string.partition(separator)
We used & as separator or Delimiter in below example :
String Functions:
There are several built-in string functions and methods that allow you to manipulate and work with strings efficiently. Here are some common string functions and methods:
strip():
- str.strip(): Removes white space from beginning & ending.
- str.lstrip(): Removes all whitespaces at the beginning of the string.
- str.rstrip(): Removes all whitespaces at the end of the string
- str.strip(‘character’): Removes character from beginning & ending.
letter case:
- str.upper() : Convert the string to uppercase.
- str.lower(): Convert the string to lowercase.
- str.capitalize(): Capitalize the first letter of the string.
- str.title(): Capitalize the first letter of each word.
str.replace(old, new):
Replace occurrences of a substring with another substring.
String Counting:
Count method is a string function that allows you to determine the number of occurrences of a specific substring within a given string. The basic syntax for the count method is as follows:
string.count(substring, start, end)
startswith():
startswith method is a string function that allows you to check if a given string starts with a specified prefix. The basic syntax for the startswith method is as follows:
string.startswith(prefix, start, end)
endswith():
endswith method is a string function that allows you to check if a given string ends with a specified suffix. The basic syntax for the endswith method is as follows:
string.endswith(suffix, start, end)
split():
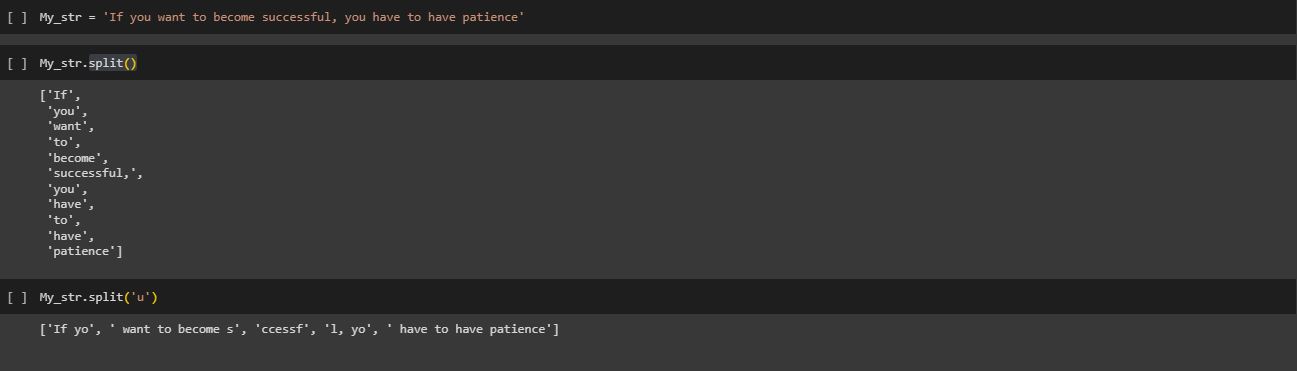
Change String to Time:
def convert_time_to_minutes(time_str):
try:
hours, minutes = time_str.split('h')
hours = int(hours.strip())
if minutes.strip('m '):
minutes = int(minutes.strip('m '))
else:
minutes = 0 # Set minutes to 0 if it's an empty string
total_minutes = hours * 60 + minutes
return total_minutes
except ValueError:
print(f"Invalid time format: {time_str}")
return None
# Example usage
time_str = "2h 40m"
converted_minutes = convert_time_to_minutes(time_str)
print(converted_minutes,'mins')
Output: 160 mins